Recently I am asked to integrate client Salesforce dataextension centre. After digging into salesforce official
website, I found there is an easy way to insert data in Salesforce data extension by calling a RESTfull API (POST /dataevents/key:{key}/rowset)
Here is the API Overview
POST https:or POST https:
[ { "keys": { "Email": "someone@example.com" }, "values": { "LastLogin": "2013-05-23T14:32:00Z", "IsActive": true, "FirstName": "John", "FollowerCount": 2, "LastName": "Smith" } } ]
However, there is an issue of this API, which only validate the email address. If email address is matching, the user's profile will be updated. Imagine if someone trying to hack the data extension or even if it's a typo. There is a potential risks that the user profile can be overwritten with wrong data.
For avoiding this, we need a bit of more code to work around.
SaleForce supports to trigger email. So instead of bringing member details to a custom form, but sending an email to the user. Here is a basic workflow
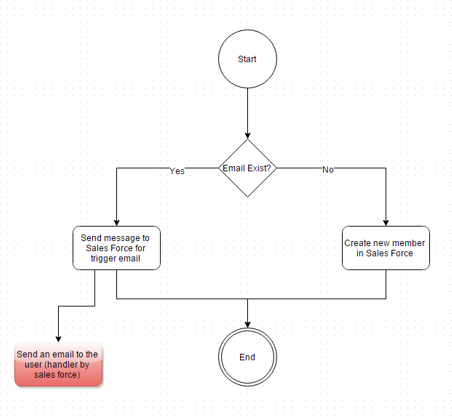
The API for triggering an email is
https://www.exacttargetapis.com/messaging/v1/messageDefinitionSends/key:SomeKey/send
In this way, member's details wont be disclosed, if the typed email address was wrong.
I am running on Sitecore CMS 7.2, and Sitecore PowerShell Extensions 3.0. After installing the powershell extension module, Publishing site is always failing. I tried re-install on a vanilla Sitecore instance, but no luck. There is still having the same error. I researched online and found it was caused by script library item, which is an known
issue. To walk around. I just need to mark the
/Sitecore/System/Module/powershell/Script Library unpublished.
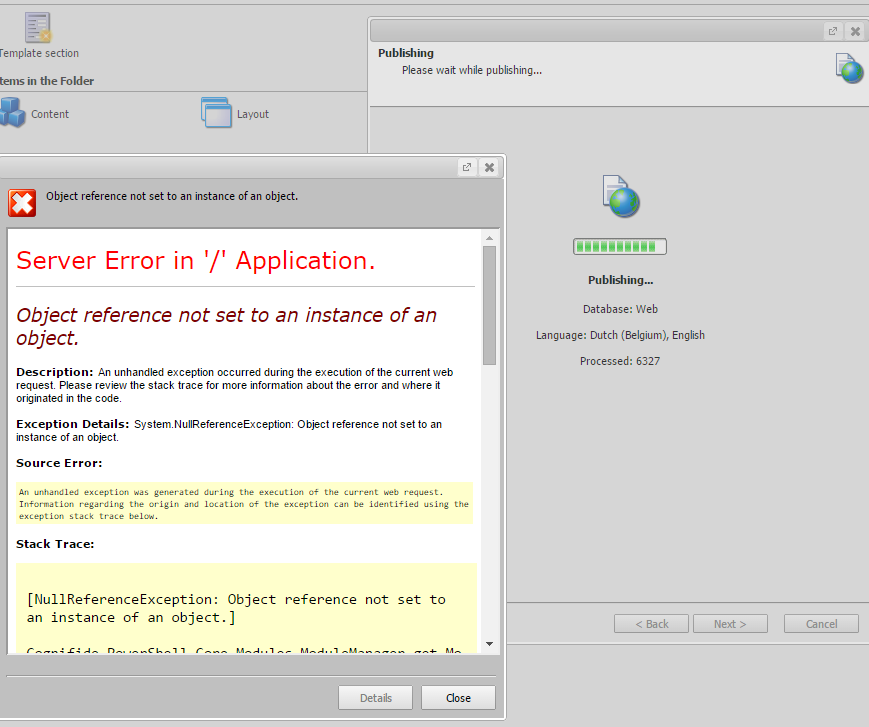
Follow this tutorial to simply setup and run your first MongoDB as service on window 8. Also you can find full details on
mongoDB official website .
- create custom mongod.conf file
Here is an example:
#where data files will reside
dbpath=C:\Program Files\MongoDB\Sitecore\data
#where the log file will be stored
logpath=C:\Program Files\MongoDB\Sitecore\log\mongo-server.log
#how verbose the server will be logging
verbose=vvvvv
mongd -f "[your mongod.conf path]" --install
This command is used for installing the mongodb as a service on your local machine with the settings written in the mongod.conf
net start mongodb
After this command, you will see a message as below
"The MongoDB service was started successfully"
NOTE: to create a MongoDB as server, you will need to run the command as Administrator, otherwise you may get an deny error.
Nowadays, WebAPI is widely used for building a RESTful service. Given the fact that Web API can automatically serialize model to JSON format, so Json becomes a very common/popular argument for WebAPI.
The question is how to effectively validate the Json object before processing your business logic. Normally, you might do something like this
if (string.isNullEmpty(object.a)){
//your logical here
}
Thinking if you have 10 properties, you will have to check 10 times and the code will be pretty nasty. So the solution below is attempted to provide an elegant way for validating the JSON object.
Solution
Implement custom
ValidationAttributeto the property. Look at the example below,
NotNullableAttribute is a customValidationAttribute which will evaluate the value. If it's null, it will throw a NoNullAllowedException.For example:
[NotNullable(ErrorMessage = "product field can not be null")]
public string Product { get; set; }
[AttributeUsage(AttributeTargets.Property, AllowMultiple = false)]
public class NotNullableAttribute : ValidationAttribute
public override bool IsValid(object value)
//place your logical here
throw new NoNullAllowedException(ErrorMessage);
If you don't want to write your own code, here is theNuGet package, or you can run the following command in the Package Manager Console.PM> Install-Package ObjectPropertyValidator
Usage of the NuGet Package
1. Step 1
Create your own classpublic class Customer
{
[NotNullable]
public string Name { get; set; }
[NotEmptyWithValidData( Pattern= @"^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,4}$")]
public string Email { get; set; }
[NotEmptyWithValidData(MaxLength =10, MinLength = 10)]
public string Phone { get; set; }
}
2.Step 2
Create your WebAPI.
[HttpPost]
public HttpResponseMessage Post(Customer customer)
{
// the argument customerwill be validated before reach your code below.
}
Conclusion
As you can see above, with the solution less code is required for data validation, and it's completely reusable.
After upgrading to Xcode 6.1, and iOS 8, I experienced network connecting issue in simulator. It worked for https requests, however, it returned an 1005 error for all http requests. I googled and tried all the suggestions that people commented on the internet[1] like "Reset simulator content", "remove ~/Library/Developer/Xcode/DeriveData", "re-install Xcode" etc. None of them was actually working for me.
For isolating the issue, I tried to run the same code on other mac and it works. Based on the most people's comments, it seems that it is due to the Xcode simulator doesn't support the network configuration somehow. So I decided to reset my Wi-Fi settings by removing extra preferred networks, and just keeping the one I normally use, and that did the magic fix.

Reference
[1]
http://stackoverflow.com/questions/25372318/error-domain-nsurlerrordomain-code-1005-the-network-connection-was-lost